Modulation
Modulation
is a mechanism to control amplitude modulation.
Modulation data is multiplied by the sound pressure amplitude.
For example, when applying Sine
modulation of , the sound pressure amplitude will be as follows, and the envelope of the positive (or negative) part of the sound pressure amplitude will follow a sine wave.
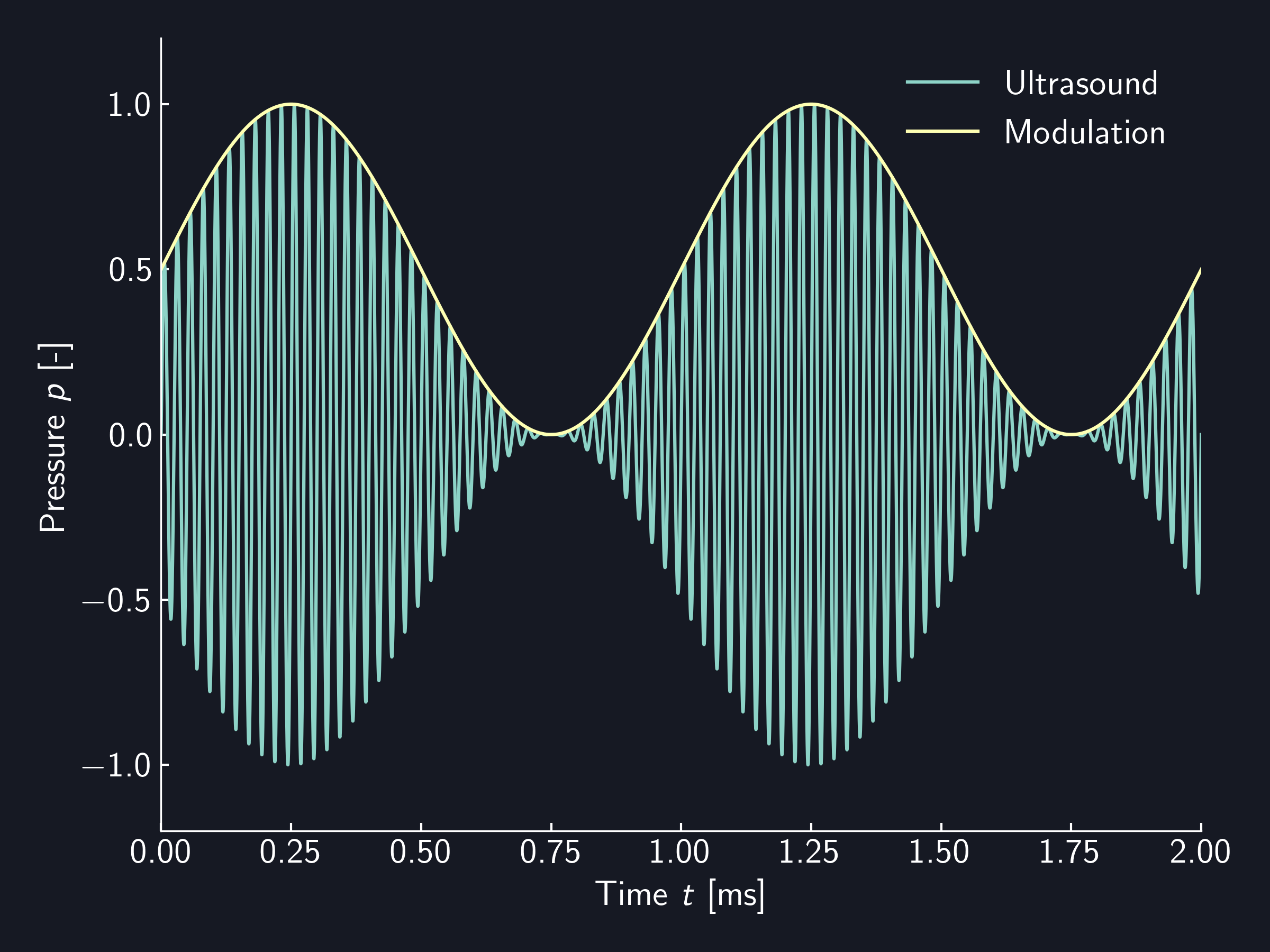
Currently, Modulation
has the following limitations.
- The buffer size is up to 32768
- 65536 when using extended mode
- The sampling rate is . Here, is a 16-bit unsigned integer greater than 0.
The SDK provides several types of Modulation
to generate AM by default.
- Static - Without modulation
- Sine - Sine wave
- Fourier - Superposition of sine waves
- Square - Square wave
- Wav - Modulation based on Wav file
- Csv - Modulation based on Csv file
- Custom - User-defined modulation
- Cache - Cache the calculation result of other
Modulation
- RadiationPressure - Apply modulation to radiation pressure instead of sound pressure
- Fir - Apply Fir filter
Common API for Modulation
Sampling Configuration
The sampling configuration of Modulation
can be obtained with sampling_config
.
use autd3::prelude::*;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let m = Sine {
freq: 150 * Hz,
option: SineOption::default(),
};
dbg!(m.sampling_config().freq()?); // -> 4kHz
Ok(())
}
#include<iostream>
#include<autd3.hpp>
#include<autd3/link/nop.hpp>
int main() {
using namespace autd3;
const auto m = Sine(150 * Hz, SineOption{});
std::cout << m.sampling_config().freq() << std::endl; // -> 4kHz
return 0; }
using AUTD3Sharp;
using AUTD3Sharp.Modulation;
using static AUTD3Sharp.Units;
var m = new Sine(freq: 150u * Hz, option: new SineOption());
Console.WriteLine(m.SamplingConfig().Freq()); // -> 4kHz
from pyautd3 import Hz
from pyautd3.modulation import Sine, SineOption
m = Sine(freq=150 * Hz, option=SineOption())
print(m.sampling_config().freq()) # -> 4kHz
Additionally, some Modulation
can optionally change the sampling configuration.
For more details on sampling configuration, refer to About Sampling Configuration.
LoopBehavior
Modulation
can control the behavior of loops.
The default is an infinite loop.
For more details, refer to Segment/LoopBehavior.