Multiple Devices
AUTD3 can connect multiple devices in a daisy-chain to form a large array. The SDK is designed to be used transparently even when multiple devices are connected.
When using multiple devices with the SDK, specify the AUTD3
structure for each connected device in order in the first argument of the Controller::open
function.
Refer to Getting Started/Hardware for hardware connection methods.
Below are the steps for connecting two devices.
Translation Only
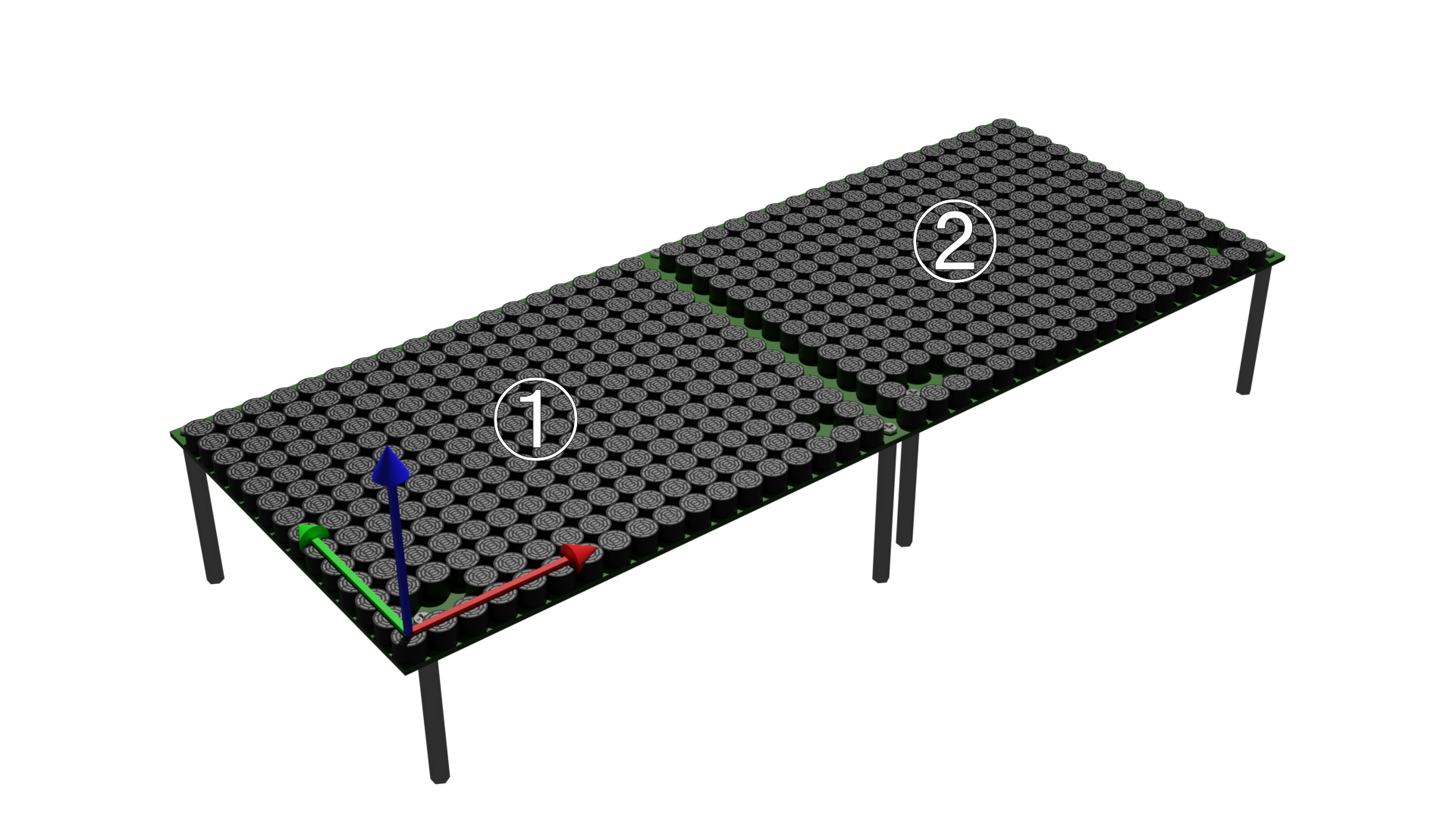
For example, if the devices are arranged and connected as shown above, with the device on the left being the first and the device on the right being the second, and the global coordinates are taken to be the same as the local coordinates of the first device, the code is as follows.
use autd3::prelude::*;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let link = autd3::link::Nop::new();
let _ =
Controller::open(
[
AUTD3 {
pos: Point3::origin(),
rot: UnitQuaternion::identity(),
},
AUTD3 {
pos: Point3::new(AUTD3::DEVICE_WIDTH, 0., 0.),
rot: UnitQuaternion::identity(),
},
],
link,
)?;
Ok(())
}
#include<chrono>
#include<autd3.hpp>
#include<autd3/link/nop.hpp>
int main() {
using namespace autd3;
link::Nop link;
Controller::open({AUTD3{
.pos = Point3::origin(),
.rot = Quaternion::Identity(),
},
AUTD3{
.pos = Point3(AUTD3::DEVICE_WIDTH, 0, 0),
.rot = Quaternion::Identity(),
}},
std::move(link));
return 0; }
using AUTD3Sharp;
using AUTD3Sharp.Link;
using AUTD3Sharp.Utils;
var link = new Nop();
Controller.Open([
new AUTD3(pos: Point3.Origin, rot: Quaternion.Identity),
new AUTD3(pos: new Point3(AUTD3.DeviceWidth, 0, 0), rot: Quaternion.Identity)
], link)
;
from pyautd3 import AUTD3, Controller
from pyautd3.link.nop import Nop
link = Nop()
Controller.open(
[
AUTD3(pos=[0.0, 0.0, 0.0], rot=[1, 0, 0, 0]),
AUTD3(pos=[AUTD3.DEVICE_WIDTH, 0.0, 0.0], rot=[1, 0, 0, 0]),
],
link,
)
Here, pos
represents the position of the device in global coordinates.
Note that AUTD3::DEVICE_WIDTH
is the width of the device (including the outer shape of the board).
Setting Global Coordinates
The origin and orientation of the global coordinates used by the SDK can be freely set by the user.
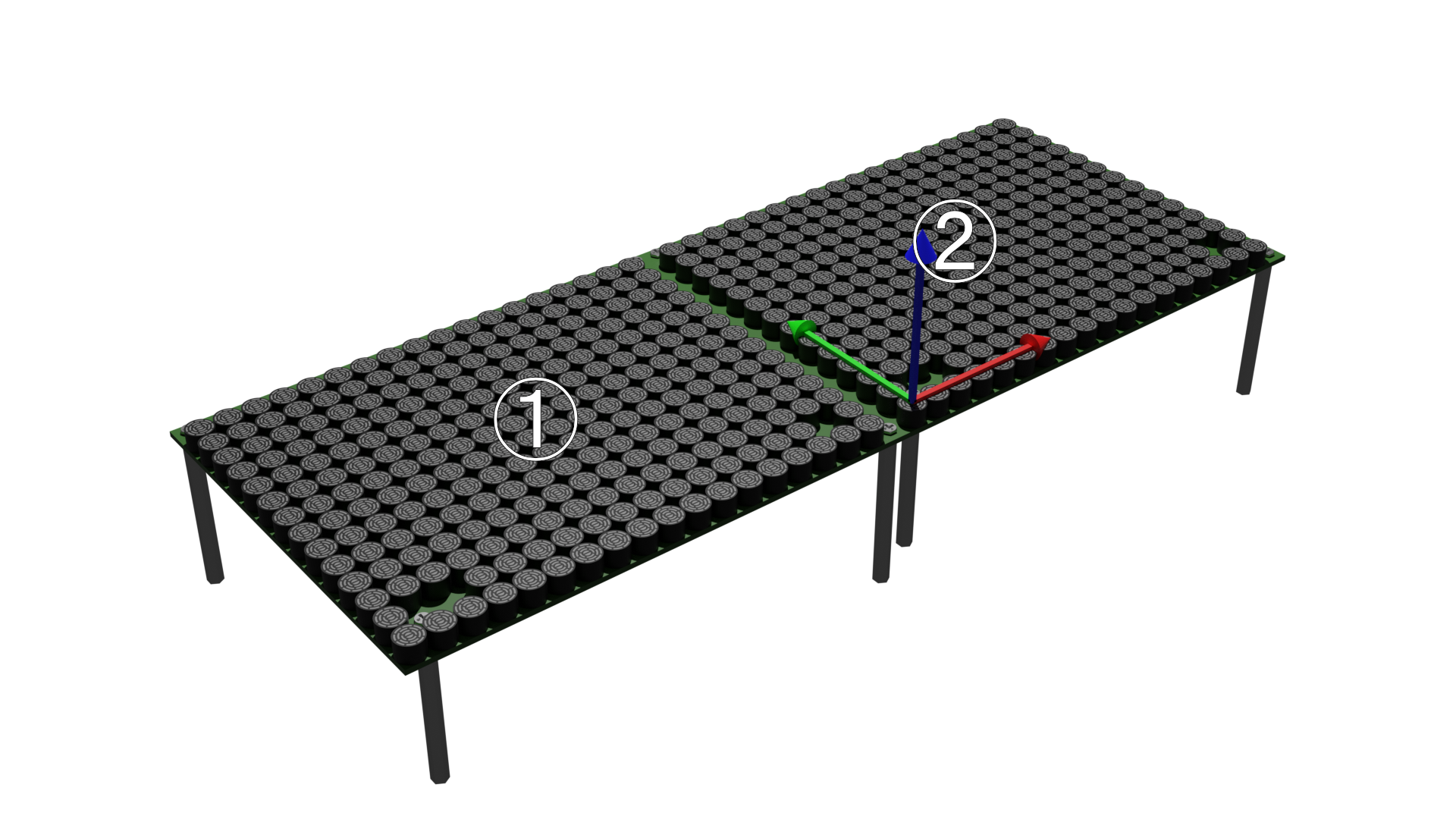
For example, if the global coordinates are taken to be the same as the local coordinates of the second device, the code is as follows.
use autd3::prelude::*;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let link = autd3::link::Nop::new();
let _ =
Controller::open(
[
AUTD3 {
pos: Point3::new(-AUTD3::DEVICE_WIDTH, 0., 0.),
rot: UnitQuaternion::identity(),
},
AUTD3 {
pos: Point3::origin(),
rot: UnitQuaternion::identity(),
},
],
link,
)?;
Ok(())
}
#include<chrono>
#include<autd3.hpp>
#include<autd3/link/nop.hpp>
int main() {
using namespace autd3;
link::Nop link;
Controller::open(
{
AUTD3{
.pos = Point3(-AUTD3::DEVICE_WIDTH, 0, 0),
.rot = Quaternion::Identity(),
},
AUTD3{
.pos = Point3::origin(),
.rot = Quaternion::Identity(),
},
},
std::move(link));
return 0; }
using AUTD3Sharp;
using AUTD3Sharp.Link;
using AUTD3Sharp.Utils;
var link = new Nop();
Controller.Open([
new AUTD3(pos: new Point3(-AUTD3.DeviceWidth, 0, 0), rot: Quaternion.Identity),
new AUTD3(pos: Point3.Origin, rot: Quaternion.Identity)
], link)
;
from pyautd3 import AUTD3, Controller
from pyautd3.link.nop import Nop
link = Nop()
Controller.open(
[
AUTD3(pos=[-AUTD3.DEVICE_WIDTH, 0.0, 0.0], rot=[1, 0, 0, 0]),
AUTD3(pos=[0.0, 0.0, 0.0], rot=[1, 0, 0, 0]),
],
link,
)
Translation and Rotation
To specify the rotation of the device, use rot
.
Rotation can be specified using Euler angles or quaternions.
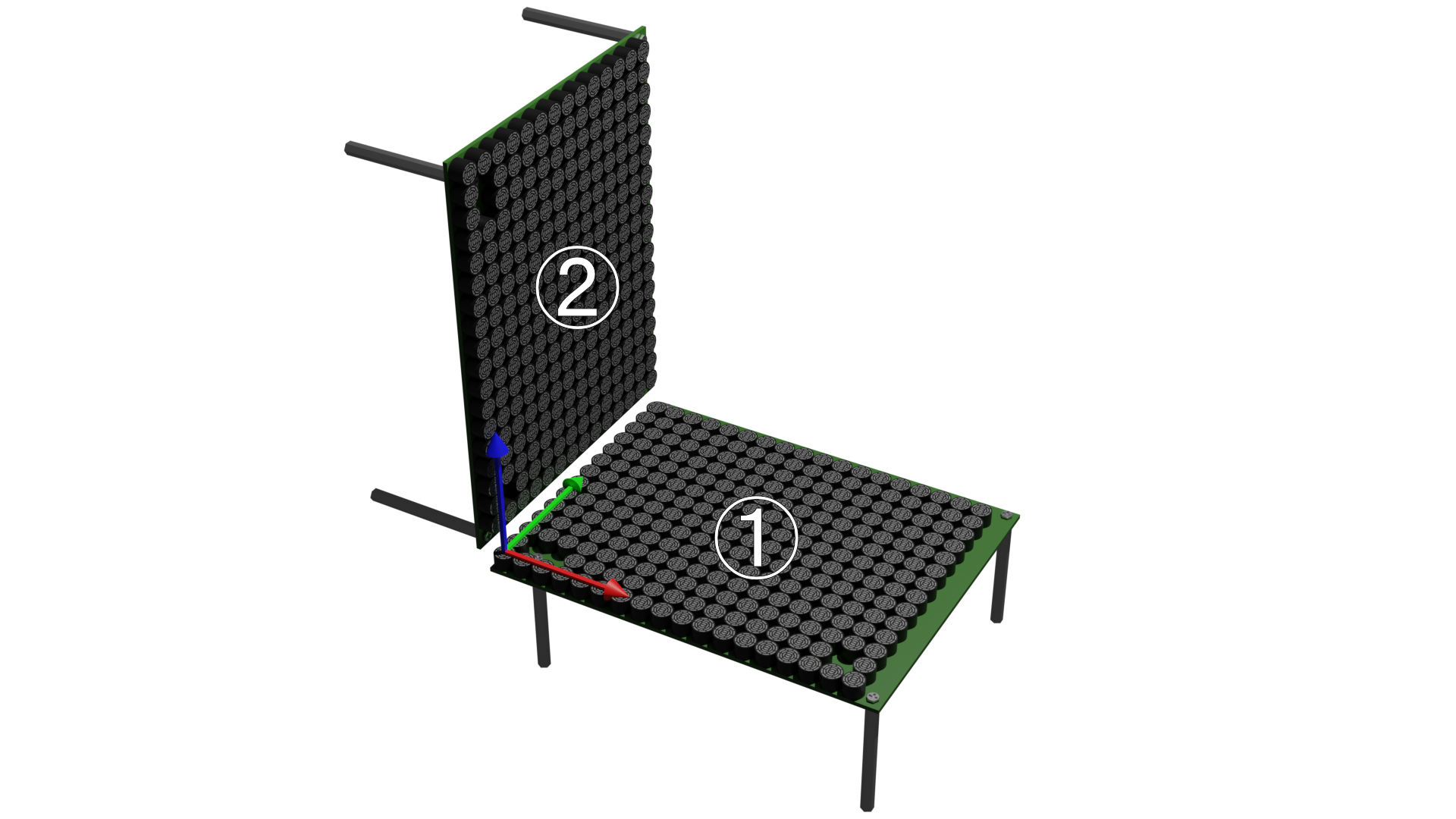
For example, if the devices are arranged as shown above, with the bottom being the first device and the left being the second device, and the global coordinates are taken to be the same as the local coordinates of the first device, the code is as follows.
use autd3::prelude::*;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let link = autd3::link::Nop::new();
let _ =
Controller::open(
[
AUTD3 {
pos: Point3::origin(),
rot: UnitQuaternion::identity(),
},
AUTD3 {
pos: Point3::new(0., 0., AUTD3::DEVICE_WIDTH),
rot: EulerAngle::ZYZ(0. * rad, PI/2.0 * rad, 0. * rad).into(),
},
],
link,
)?;
Ok(())
}
#include<chrono>
#include<autd3.hpp>
#include<autd3/link/nop.hpp>
int main() {
using namespace autd3;
link::Nop link;
Controller::open({AUTD3{
.pos = Point3::origin(),
.rot = Quaternion::Identity(),
},
AUTD3{
.pos = Point3(0, 0, AUTD3::DEVICE_WIDTH),
.rot = EulerAngles::ZYZ(0. * rad, pi / 2.0 * rad,
0. * rad),
}},
std::move(link));
return 0; }
using System;
using AUTD3Sharp;
using AUTD3Sharp.Link;
using AUTD3Sharp.Utils;
using static AUTD3Sharp.Units;
var link = new Nop();
Controller.Open([
new AUTD3(pos: Point3.Origin, rot: Quaternion.Identity),
new AUTD3(
pos: new Point3(0, 0, AUTD3.DeviceWidth),
rot: EulerAngles.Zyz(0 * rad, MathF.PI / 2 * rad, 0 * rad))
], link)
;
import numpy as np
from pyautd3 import AUTD3, Controller, EulerAngles, Nop, rad
link = Nop()
Controller.open(
[
AUTD3(pos=[0.0, 0.0, 0.0], rot=[1.0, 0.0, 0.0, 0.0]),
AUTD3(
pos=[0.0, 0.0, AUTD3.DEVICE_WIDTH],
rot=EulerAngles.ZYZ(0 * rad, np.pi / 2 * rad, 0 * rad),
),
],
link,
)
NOTE: Only the Rust version supports all 12 types of Euler angles. Other languages support only XYZ and ZYZ.