Controller
The followings are introductino of APIs in Controller
class.
Force fan
AUTD3 device has a fan, and it has three fan modes: Auto, Off, and On.
In Auto mode, the temperature monitoring IC monitors the temperature of the IC, and when it exceeds a certain temperature, the fan starts automatically. In Off mode, the fan is always off, and in On mode, the fan is always on.
The fan mode is switched by the jumper switch next to the fan. As shown in the figure below, the fan side is shorted to switch to Auto, the center is Off, and the right is On.
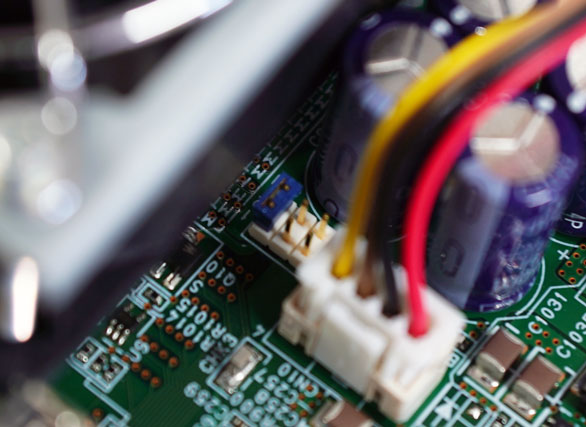
You can force the fan to start in Auto mode by ForceFan
.
use autd3::prelude::*;
#[allow(unused_variables)]
fn main() -> Result<(), Box<dyn std::error::Error>> {
let mut autd = Controller::builder([AUTD3::new(Point3::origin())]).open(autd3::link::Nop::builder())?;
autd.send(ForceFan::new(|_dev| true))?;
Ok(())
}
#include<chrono>
#include<autd3.hpp>
#include<autd3/link/nop.hpp>
int main() {
auto autd =
autd3::ControllerBuilder({autd3::AUTD3(autd3::Point3::origin())}).open(autd3::link::Nop::builder());
autd.send(autd3::ForceFan([](const auto&) { return true; }));
return 0; }
using AUTD3Sharp;
using AUTD3Sharp.Link;
using AUTD3Sharp.Utils;
using var autd = Controller.Builder([new AUTD3(Point3.Origin)]).Open(Nop.Builder());
autd.Send(new ForceFan(_ => true));
from pyautd3 import ForceFan
from pyautd3 import Controller, AUTD3
from pyautd3.link.audit import Audit
autd = Controller.builder([AUTD3([0.0, 0.0, 0.0])]).open(Audit.builder())
autd.send(ForceFan(lambda _: True))
fpga_state
Get the FPGA status.
Before using this, you need to configure reads FPGA info flag by ReadsFPGAState
.
use autd3::prelude::*;
#[allow(unused_variables)]
fn main() -> Result<(), Box<dyn std::error::Error>> {
let mut autd = Controller::builder([AUTD3::new(Point3::origin())]).open(autd3::link::Nop::builder())?;
autd.send(ReadsFPGAState::new(|_dev| true))?;
let info = autd.fpga_state()?;
Ok(())
}
#include<autd3.hpp>
#include<autd3/link/nop.hpp>
int main() {
auto autd =
autd3::ControllerBuilder({autd3::AUTD3(autd3::Point3::origin())}).open(autd3::link::Nop::builder());
autd.send(autd3::ReadsFPGAState([](const auto&) { return true; }));
const auto info = autd.fpga_state();
return 0; }
using AUTD3Sharp;
using AUTD3Sharp.Link;
using AUTD3Sharp.Utils;
using var autd = Controller.Builder([new AUTD3(Point3.Origin)]).Open(Nop.Builder());
autd.Send(new ReadsFPGAState(_ => true));
var info = autd.FPGAState;
from pyautd3 import Controller, AUTD3, ReadsFPGAState
from pyautd3.link.audit import Audit
autd = Controller.builder([AUTD3([0.0, 0.0, 0.0])]).open(Audit.builder())
autd.send(ReadsFPGAState(lambda _: True))
info = autd.fpga_state
You can get the following information about the FPGA.
- thermal sensor for fan control is asserted or not
send
Send the data to the device.
You can send a single or two data at the same time.
Timeout
You can specify the timeout time with with_timeout
.
If you omit this, the timeout time set by Link will be used.
use std::time::Duration;
use autd3::prelude::*;
#[allow(unused_variables)]
fn main() -> Result<(), Box<dyn std::error::Error>> {
let mut autd = Controller::builder([AUTD3::new(Point3::origin())]).open(autd3::link::Nop::builder())?;
let m = Static::new();
let g = Null::new();
autd.send((m, g).with_timeout(Some(Duration::from_millis(20))))?;
Ok(())
}
#include<chrono>
#include<autd3.hpp>
#include<autd3/link/nop.hpp>
int main() {
auto autd =
autd3::ControllerBuilder({autd3::AUTD3(autd3::Point3::origin())}).open(autd3::link::Nop::builder());
autd3::Static m;
autd3::Null g;
autd.send((m, g).with_timeout(std::chrono::milliseconds(20)));
return 0; }
using AUTD3Sharp;
using AUTD3Sharp.Link;
using AUTD3Sharp.Gain;
using AUTD3Sharp.Modulation;
using AUTD3Sharp.Utils;
using AUTD3Sharp.Driver.Datagram;
using var autd = Controller.Builder([new AUTD3(Point3.Origin)]).Open(Nop.Builder());
var m = new Static();
var g = new Null();
autd.Send((m, g).WithTimeout(Duration.FromMillis(20)));
# mypy: ignore-errors
from pyautd3 import Controller, AUTD3, Static, Null, Duration
from pyautd3.link.audit import Audit
autd = Controller.builder([AUTD3([0.0, 0.0, 0.0])]).open(Audit.builder())
m = Static()
g = Null()
autd.send((m, g).with_timeout(Duration.from_millis(20)))
If the timeout time is greater than 0, the send
function waits until the sent data is processed by the device or the specified timeout time elapses.
If it is confirmed that the sent data has been processed by the device, the send
function returns true
, otherwise it returns false
.
If the timeout time is 0, the send
function does not check whether the sent data has been processed by the device or not.
If you want to data to be sent surely, it is recommended to set this to an appropriate value.
Clear
You can clear the flags and Gain
/Modulation
data in the device by sending Clear
data.
group
You can group the devices by using group
function, and send different data to each group.
use autd3::prelude::*;
#[allow(unused_variables)]
fn main() -> Result<(), Box<dyn std::error::Error>> {
let mut autd = Controller::builder([AUTD3::new(Point3::origin()), AUTD3::new(Point3::origin())]).open(autd3::link::Nop::builder())?;
let x = 0.;
let y = 0.;
let z = 0.;
autd.group(|dev| match dev.idx() {
0 => Some("focus"),
1 => Some("null"),
_ => None,
})
.set("null", Null::new())?
.set("focus", Focus::new(Point3::new(x, y, z)))?
.send()?;
Ok(())
}
#include<chrono>
#include<autd3.hpp>
#include<autd3/link/nop.hpp>
int main() {
auto autd =
autd3::ControllerBuilder({autd3::AUTD3(autd3::Point3::origin())}).open(autd3::link::Nop::builder());
const auto x = 0.0;
const auto y = 0.0;
const auto z = 0.0;
autd.group([](const autd3::Device& dev) -> std::optional<const char*> {
if (dev.idx() == 0) {
return "null";
} else if (dev.idx() == 1) {
return "focus";
} else {
return std::nullopt;
}
})
.set("null", autd3::gain::Null())
.set("focus", autd3::gain::Focus(autd3::Point3(x, y, z)))
.send();
return 0; }
using AUTD3Sharp;
using AUTD3Sharp.Link;
using AUTD3Sharp.Gain;
using AUTD3Sharp.Modulation;
using AUTD3Sharp.Utils;
using var autd = Controller.Builder([new AUTD3(Point3.Origin)]).Open(Nop.Builder());
var x = 0.0f;
var y = 0.0f;
var z = 0.0f;
autd.Group(dev =>
{
return dev.Idx switch
{
0 => "null",
1 => "focus",
_ => null
};
})
.Set("null", new Null())
.Set("focus", new Focus(new Point3(x, y, z)))
.Send();
from pyautd3.gain import Focus, Null
from pyautd3 import Controller, AUTD3, Device
from pyautd3.link.audit import Audit
autd = Controller.builder([AUTD3([0.0, 0.0, 0.0])]).open(Audit.builder())
x = 0.0
y = 0.0
z = 0.0
def grouping(dev: Device) -> str | None:
if dev.idx == 0:
return "null"
if dev.idx == 1:
return "focus"
return None
autd.group(grouping).set("null", Null()).set("focus", Focus([x, y, z])).send()
Unlike gain::Group
, you can use any data that can be sent with send
as a value.
However, you can only group by device.
NOTE: This sample uses a string as a key, but you can use anything that can be used as a key for HashMap.